你有没有想过,在Unity游戏中,如何让玩家在每次离开时,都能带着满满的回忆再次归来呢?没错,这就是我们今天要聊的——Unity游戏存档!想象玩家在游戏中历经千辛万苦,终于通关了某个关卡,突然,电源“啪”的一下断了,玩家的心都碎了。别担心,有了存档,这一切都不是问题!
存档,让回忆永不消失
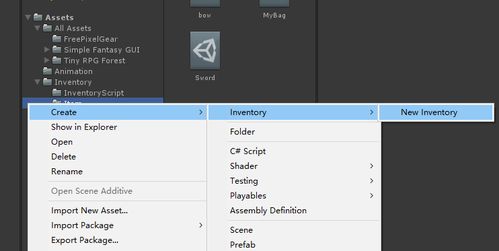
存档,顾名思义,就是将游戏中的数据保存下来,以便玩家下次继续游戏时能够从上次离开的地方继续。在Unity中,实现存档的方式有很多,下面我们就来一一揭晓。
1. PlayerPrefs:简单易用的小帮手

PlayerPrefs是Unity自带的一个小工具,它就像一个电子记事本,可以用来存储一些简单的数据,比如玩家的名字、音量设置等。使用PlayerPrefs非常简单,就像在记事本上写写画画一样。
```csharp
PlayerPrefs.SetInt(\Score\, 100); // 存储分数
PlayerPrefs.GetString(\Name\, \张三\); // 存储玩家名字
PlayerPrefs.SetFloat(\Volume\, 0.5f); // 存储音量大小
PlayerPrefs.Save(); // 保存数据
虽然PlayerPrefs功能强大,但它只能存储int、float和string类型的数据,对于更复杂的数据结构,我们就需要借助其他方法了。
2. 序列化:让复杂数据也能存档
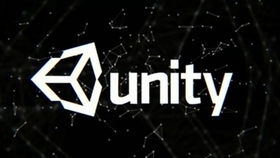
序列化是将对象转换成可以存储或传输的格式的过程。在Unity中,我们可以使用C的序列化功能,将游戏中的对象转换成JSON或XML格式,然后保存到文件中。
```csharp
using System;
using System.IO;
using UnityEngine;
[System.Serializable]
public class SaveData
public int health;
public Vector3 position;
public class SaveSystem : MonoBehaviour
public void SaveGame(SaveData data)
{
string json = JsonUtility.ToJson(data);
File.WriteAllText(Application.persistentDataPath + \/savefile.json\, json);
}
public SaveData LoadGame()
{
string path = Application.persistentDataPath + \/savefile.json\;
if (File.Exists(path))
{
string json = File.ReadAllText(path);
return JsonUtility.FromJson
}
else
{
return new SaveData();
}
}
使用序列化,我们可以将游戏中的任何对象保存下来,包括角色、道具、关卡等。
3. XML和JSON:存储数据的利器
XML和JSON是两种常用的数据存储格式,它们具有可读性强、易于读写等优点。
XML示例:
```csharp
using System;
using System.Xml;
using UnityEngine;
[System.Serializable]
public class SaveData
public int health;
public Vector3 position;
public class SaveSystem : MonoBehaviour
public void SaveGame(SaveData data)
{
XmlDocument xmlDoc = new XmlDocument();
XmlElement root = xmlDoc.CreateElement(\SaveData\);
XmlElement health = xmlDoc.CreateElement(\Health\);
health.InnerText = data.health.ToString();
root.AppendChild(health);
XmlElement position = xmlDoc.CreateElement(\Position\);
position.SetAttribute(\x\, data.position.x.ToString());
position.SetAttribute(\y\, data.position.y.ToString());
position.SetAttribute(\z\, data.position.z.ToString());
root.AppendChild(position);
xmlDoc.AppendChild(root);
xmlDoc.Save(Application.persistentDataPath + \/savefile.xml\);
}
public SaveData LoadGame()
{
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(Application.persistentDataPath + \/savefile.xml\);
XmlElement root = xmlDoc.DocumentElement;
SaveData data = new SaveData();
data.health = int.Parse(root.SelectSingleNode(\Health\).InnerText);
data.position = new Vector3(
float.Parse(root.SelectSingleNode(\Position\).GetAttribute(\x\)),
float.Parse(root.SelectSingleNode(\Position\).GetAttribute(\y\)),
float.Parse(root.SelectSingleNode(\Position\).GetAttribute(\z\))
);
return data;
}
JSON示例:
```csharp
using System;
using System.IO;
using UnityEngine;
[System.Serializable]
public class SaveData
public int health;
public Vector3 position;
public class SaveSystem : MonoBehaviour
public void SaveGame(SaveData data)
{
string json = JsonUtility.ToJson(data);
File.WriteAllText(Application.persistentDataPath + \/savefile.json\, json);
}
public SaveData LoadGame()
{
string path = Application.persistentDataPath + \/savefile.json\;
if (File.Exists(path))
{
string json = File.ReadAllText(path);
return JsonUtility.FromJson
}
else
{
return new SaveData();
}
}